
Introduction
The Revers.io API is based on REST principle, using JSON-encoded bodies, and returning json-encoded responses.
We use standard HTTP verbs and response codes.
It is designed for Server to Server calls, and such should not be used from client applications directly.
Authenticating with the API
Using any API requires a Subscription Key and an API Token.
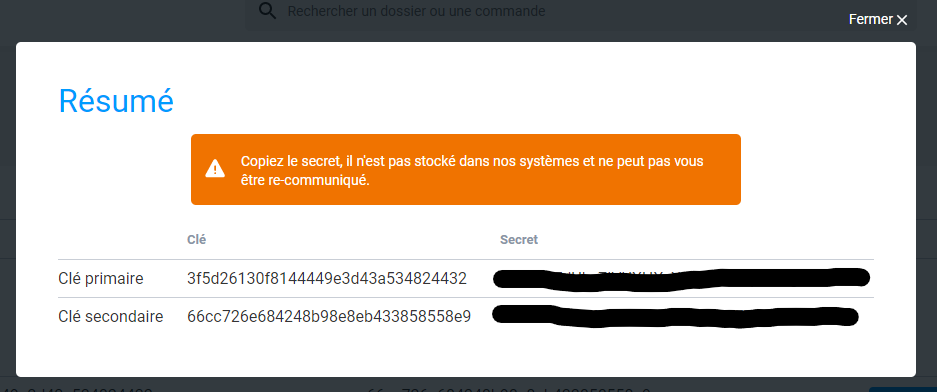
Obtaining a Subscription Key and Api Token
This operation is only necessary the first time you use our APIs or when you need to change your API token.
Connect to the API key management page and create a new application in "Import by API" menu. This will provide you with two Subscription Key / Api Token pairs (to allow for easy rotations).
Obtaining a JWT Token
In order to use any API, you need to acquire a JWT token using your Subscription Key / Api Token pair.
Note that such a token has a validity of one hour and should be regenerated accordingly. You can find the expiration date of a token by decoding the token and use the exp claim.
To obtain said token, call the Get token API with the proper Headers set.
curl https://customer-api.revers.io/api/v1/token
-H "Ocp-Apim-Subscription-Key: {subscription key}"
-H "Ocp-Apim-Secret-Value: {secret value}"
Pass your subscription key using the Ocp-Apim-Subscription-Key header and your secret using Ocp-Apim-Secret-Value header.
Under no circumstances should you send your Api Key to another API.
curl https://customer-api.revers.io/api/v1/orders/{orderReference}/status
-H "Ocp-Apim-Subscription-Key: {subscription key}"
-H "Authorization: Bearer xxxxxxxxxxxxxxxxxxxxxxxx"
Using a JWT token
For any subsequent API call, authentification is insured by usage of the JWT Token as a Bearer Token in the Authorization header as well as the Subscription Key.
For clarity, we will omit these two headers in the next paragraphs of this article.
Import Catalog & Order
To trigger any AfterSales File creation, we need to enter Order data in the platform, referencing the Product Catalog.
In this section, you will learn how you can create a Model in Catalog, representing the sold Product, and how to use it to create your first Order.Create a Model
Let's create our first Model, representing a Product.
GET https://customer-api.revers.io/api/v1/catalog/modelTypes
{
"value": [
{
"id": "00000000-9999-0000-0000-000000000001",
"key": "0001",
"cultureCode": "fr",
"label": "Smartphone",
"description": ""
}
]
}
Retrieve ModelType
When configuring the platform, you will define your ModelTypes with the delivery team. You will need to get the corresponding ModelType Id when creating a Model. The list of ModelTypes can be retrieved through this call.
The ModelType enables to setup the right rules for your after sales scenario.
POST https://customer-api.revers.io/api/v1/catalog/brands
{
"name": "Fairphone"
}
{
"value":
{
"id": "00000000-0000-0000-0000-000000000001"
}
}
Create Brand
When configuring the platform, you will define your ModelTypes with our delivery team. You will need to get the corresponding ModelType Id when creating a Model. The list of ModelTypes can be retrieved through this call.
POST https://customer-api.revers.io/api/v1/catalog/models
{
"label": "Fairphone 3+ 64GB",
"sKU": "123456",
"brandId": "00000000-0000-0000-0000-000000000001",
"modelTypeId": "00000000-9999-0000-0000-000000000001",
"state": "New",
"weight": 0.188,
"dimension":
{
"lengthInCm": 16,
"widthInCm": 7,
"heightInCm": 1
}
}
{
"value":
{
"id": "10000000-5555-0000-0000-000000000001",
[...]
}
}
Create Model
Let's create our first Model, representing a Product.
It should have
A unique id called SKU, and a label (visible from users)
An association with a Brand through the brandId
An association with a Model Type (Category) through the modelTypeId
Some information relative to dimension and weight, and state of the model